Using the PWM Output with Talon SRX¶
The Canandcoder is very easy to integrate with Talon SRX motor controllers. Outputting a duty cycle PWM signal, it enables closed loop control on the SRX.
Warning
When using the Helium Canandcoder over PWM with a TalonSRX, ReduxLib and Alchemist will not be able to configure the device. Use Phoenix Tuner/Phoenix Tuner X to configure the device via the TalonSRX instead!
Hardware¶
The Canandcoder, by default, is set up to output in REV Spark Max mode. To convert the encoder to CTRE Talon compatible output, you have to disconnect the MAX output, and bridge the SRX output.
The MAX output is enabled via a small trace between the MAX solder pads. The trace is highlighted in red in the below image.
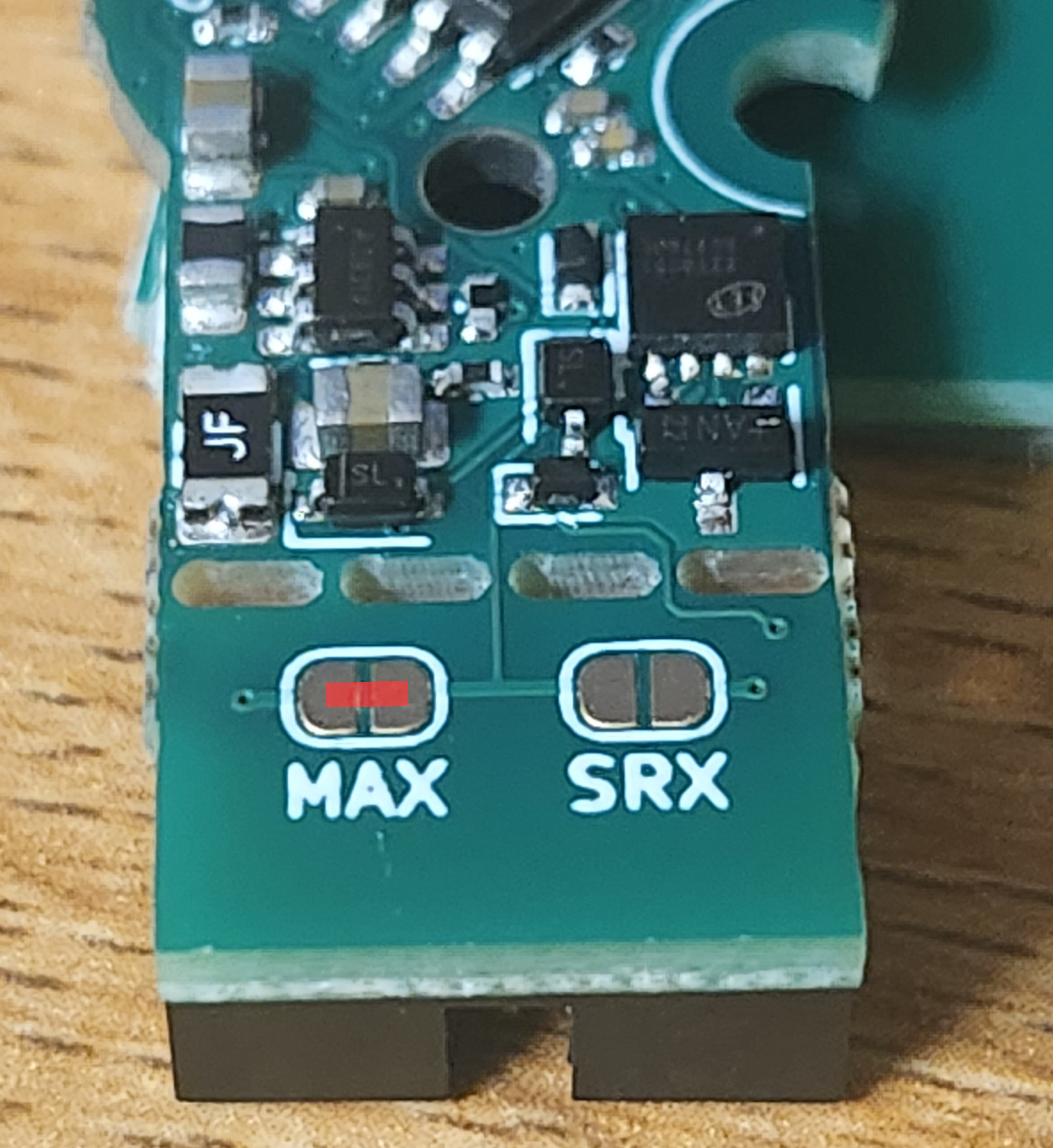
To disconnect it, use a small knife to cut the trace to disconnect it. Cut along the red line in the image below.
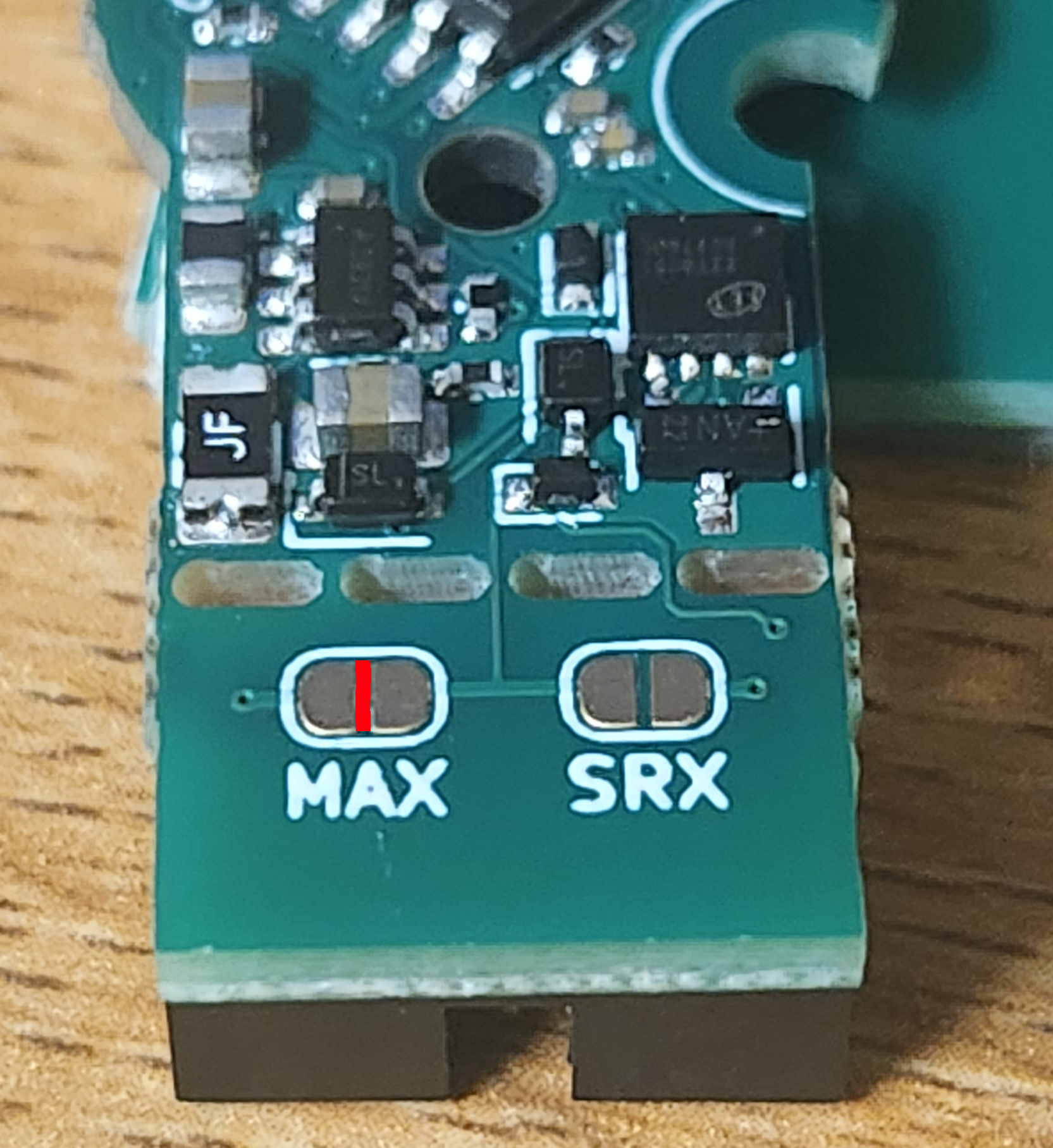
After that, bridge the solder pad marked “SRX”. The Canandcoder will now output in Talon compatible mode. Make sure not to bridge both the Spark MAX and Talon SRX pads at the same time, as this could damage your motor controller.
You can now directly connect the 10 pin connector on the Canandcoder to the 10 pin data port on the Talon SRX.
Software¶
The Canandcoder outputs a duty cycle PWM signal corresponding to the absolute angle in a 2049µs period, with a 1us pulse being 0 and a 2048µs pulse being 2047/2048ths of a rotation (~359.82deg). Between these two deadbands is a pulse resolution of 0.125 µs, for 14 bits of general resolution with a 0.18deg deadband at the extremes.
Pressing the zero button on the Canandcoder will set the currently read position as the new zero point.
This can be used to quickly rezero a mechanism, such as a sweve module, without a computer.
If the encoder is additionally connected over CAN, manipulating the absolute position via setAbsPosition()
or inverting the
direction through either the ReduxLib API or Alchemist will also change the PWM output accordingly, overriding the
offset saved by the button press.
Code Example - Java, Phoenix 5¶
TalonSRX talonsrx = new TalonSRX(1);
// configure the device to use a PWM position input
talonsrx.configSelectedFeedbackSensor(FeedbackDevice.PulseWidthEncodedPosition);
// Zeros the relative sensor position SRX-side.
// note that this is not the same as zeroing the value by pressing the onboard button!
talonsrx.setSelectedSensorPosition(0);
// Returns relative position. one rotation is 4096 units.
talonsrx.getSelectedSensorPosition();
// Absolute position can be derived from getPulseWidthRiseToFallUs(), which will range from 0 to 2047 inclusive. This will include Canandcoder-local offsets like zeroing with the onboard button.
talonsrx.getSensorCollection().getPulseWidthRiseToFallUs();
/**
* TODO: Set up closed loop configuration here
* For more information, see CTRE's documentation
*/
//Rotate 10 rotations, each rotation being 4096 units
talonsrx.set(ControlMode.Position, 10 * 4096);
For more information, refer to Cross The Road Electronics’s documentation for specifics on implementing absolute encoders with the Talon SRX. As noted in this documentation, using the PWM absolute output means kSensorUnitsPerRotation is 4096.